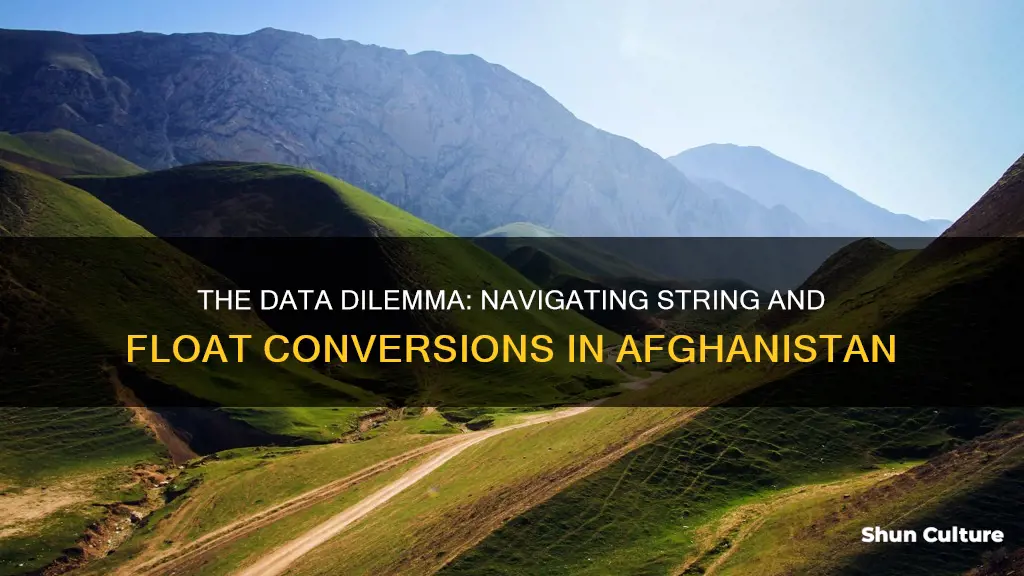
The error message could not convert string to float is a common issue in programming, particularly in Python. This error occurs when a program tries to convert a string into a floating-point number, but the conversion fails due to invalid characters or formatting in the string. In the context of Afghanistan, this error might occur if a programmer is working with data related to the country Afghanistan and attempts to convert a string value containing the country's name or other related data into a floating-point number.
Characteristics | Values |
---|---|
Error Message | ValueError: could not convert string to float |
Programming Language | Python |
Possible Causes | Incorrect data format, missing data, incompatible data types |
Suggested Solutions | Data cleaning, data transformation, error handling |
What You'll Learn
Using Pandas to convert strings to floats
There are two methods to convert strings to floats in a Pandas DataFrame: the `DataFrame.astype()` function and the `pandas.to_numeric()` function. The former allows you to convert Pandas objects to any data type, while the latter is used to convert into integer or float data types only.
Using `DataFrame.astype()`
The `DataFrame.astype()` method is used to cast a Pandas object to a specified data type. Its syntax is:
`DataFrame.astype(self: ~ FrameOrSeries, dtype, copy: bool = True, errors: str = 'raise')'
Here's an example of how to use it to convert a column from string to float:
Python
Importing pandas library
Import pandas as pd
Creating a dictionary
Data = {'Year': ['2016', '2017', '2018'],
'Inflation Rate': ['4.47', '5', '3.2']}
Create a dataframe
Df = pd.DataFrame(Data)
Converting each value of the column to a float
Df ['Inflation Rate'] = df ['Inflation Rate'].astype(float)
Show the dataframe
Print(df)
Show the data types
Print(df.dtypes)
Using `pandas.to_numeric()`
The `pandas.to_numeric()` function converts the argument to a numeric type (integer or float). Its syntax is:
`pandas.to_numeric(arg, errors='raise', downcast=None)
Here's an example of how to use it to convert a column from string to float:
Python
Importing pandas library
Import pandas as pd
Creating a dictionary
Data = {'Year': ['2016', '2017', '2018'],
'Inflation Rate': ['4.47', '5', '3.2']}
Create a dataframe
Df = pd.DataFrame(Data)
Converting each value of the column to a float
Df ['Inflation Rate'] = pd.to_numeric(df ['Inflation Rate'])
Show the dataframe
Print(df)
Show the data types
Print(df.dtypes)
Note that if the string column contains non-numeric values, the conversion will raise a `ValueError'. You can handle this by using `errors='coerce' to convert the value at this position to NaN.
Converting multiple or all columns
You can also use the `astype()` and `to_numeric()` functions to convert multiple or all columns in a DataFrame from string to float. To convert multiple columns, pass a dictionary of column names and data types to the `astype()` method:
Python
Convert multiple columns
Df = df.astype({'Fee':'float','Discount':'float'})
To convert all columns to floats, simply call the `astype()` method on the DataFrame without specifying any columns:
Python
Convert all columns to floats
Df = df.astype(float)
Scenarios for converting strings to floats in a Pandas DataFrame
Scenario 1: Numeric values stored as strings
In this scenario, we have a DataFrame with a 'Price' column that contains numeric values stored as strings. We want to convert these values into floats.
Python
Import pandas as pd
Data = {
"Product": ["aaa", "bbb", "ccc", "ddd", "eee"],
"Price": ["250", "270", "350", "400", "550"],
}
Df = pd.DataFrame(data)
Convert the 'Price' column to float
Df ["Price"] = df ["Price"].astype(float)
Alternatively, you can use the apply(float) method
Df ["Price"] = df ["Price"].apply(float)
Scenario 2: Convert strings to floats under an entire DataFrame
In this scenario, we have a DataFrame with three columns, where all the values are stored as strings. We want to convert all the values into floats.
Python
Import pandas as pd
Data = {
"Price_1": ["300", "750", "600", "770", "920"],
"Price_2": ["250", "270", "950", "580", "410"],
"Price_3": ["530", "480", "420", "290", "830"],
}
Df = pd.DataFrame(data)
Convert all columns to floats
Df = df.astype(float)
The Human Cost of War: Counting Afghanistan's Amputees
You may want to see also
Using the 'apply' function to convert
The apply function in Python is used to apply a function along an axis of the DataFrame. It is useful when you need to transform your data using a custom function.
Let's consider an example where we have a DataFrame with a column of strings representing floating-point numbers, and we want to convert these strings to floats. We can use the apply function with a lambda function to achieve this:
Python
Import pandas as pd
Create a DataFrame with a column of strings
Data = pd.DataFrame({'values': ['1.23', '4.56', '7.89']})
Apply the lambda function to convert strings to floats
Data['values'] = data['values'].apply(lambda x: float(x))
Print(data)
The output will be:
Values
0 1.23
1 4.56
2 7.89
In this example, we first import the pandas library and create a DataFrame called data with a column 'values' containing strings '1.23', '4.56', and '7.89'. Then, we use the apply function on the 'values' column and pass a lambda function that takes each string x and converts it to a float using the float() function. Finally, we print the resulting DataFrame, which now has floating-point numbers in the 'values' column.
The apply function is a versatile tool in Python that allows you to apply custom functions to your data, making it easier to perform data transformations and calculations.
Veterans Returning to Afghanistan: A Complex Journey
You may want to see also
Using 'pd.to_numeric' to convert
Pandas is a package that makes importing and analysing data much easier. It has a function called `pd.to_numeric()` that is used to convert arguments to a numeric type. This function is particularly useful for data cleaning and preparation tasks in data analysis and manipulation workflows, aiding in the transformation of heterogeneous data into a consistent numeric format.
The syntax for the `pd.to_numeric()` function is:
Python
Pandas.to_numeric(arg, errors='raise', downcast=None)
Here, `arg` is the scalar, list, tuple, 1-D array, or Series that you want to be converted. The `errors` parameter determines how the function handles invalid parsing:
- If set to 'raise', invalid parsing will raise an exception.
- If set to 'coerce', invalid parsing will be set as NaN.
- If set to 'ignore', invalid parsing will return the input.
The `downcast` parameter is used to downcast the resulting data to the smallest numerical dtype possible. If set to None, the default return dtype is `float64` or `int64` depending on the data supplied. The available options for `downcast` are:
- 'integer' or 'signed': smallest signed int dtype (min.: `np.int8`)
- 'unsigned': smallest unsigned int dtype (min.: `np.uint8`)
- 'float': smallest float dtype (min.: `np.float32`)
Python
Example 1: Convert a series to numeric
Ser2 = pd.to_numeric(ser)
Example 2: Convert a Series to float
Ser2 = pd.to_numeric(ser, downcast='float')
Example 3: Convert a Series to integer
Ser2 = pd.to_numeric(ser, downcast='signed')
Example 4: Using errors='ignore'
Ser = pd.Series(['Marks', 22, 38.5, 45, -32])
Ser2 = pd.to_numeric(ser, errors='ignore')
Example 5: Using errors='coerce'
Ser = pd.Series(['Marks', 22, 38.5, 45, -32])
Ser2 = pd.to_numeric(ser, errors='coerce')
Note that `pd.to_numeric()` does not work if there is a string in the column. If you encounter a `ValueError` due to the presence of strings, you can use the errors='coerce' parameter to replace all non-numeric values with NaN.
The Great Afghan Exodus: A Nation in Flight
You may want to see also
Using 'astype' to convert
The `astype()` function in pandas is a versatile tool that allows you to change the data type of your DataFrame. It can be used to convert a pandas Series or DataFrame from one data type to another. This is particularly useful when you need to perform operations that are specific to a certain data type.
The basic syntax for using `astype()` is:
Python
Dataframe['column_name'] = df['column_name'].astype(new_data_type)
Here, `dataframe` is the name of your DataFrame, `column_name` is the name of the column you want to change the data type of, and `new_data_type` is the data type you want to convert to.
For example, let's say you have a DataFrame `df` with a column `'A'` that contains strings, and you want to convert these strings to integers. You can use the following code:
Python
Import pandas as pd
Df = pd.DataFrame({'A': ['1', '2', '3']})
Df['A'] = df['A'].astype(int)
Print(df['A'])
The output will be:
0 1
1 2
2 3
Name: A, dtype: int64
You can also use `astype()` to convert to more complex data types, such as datetime or categorical data types. For example, if you have a DataFrame with a column of strings representing dates, you can use `astype()` to convert these strings into datetime objects:
Python
Import pandas as pd
Df = pd.DataFrame({'Date': ['2021-01-01', '2021-02-01', '2021-03-01']})
Df['Date'] = df['Date'].astype('datetime64 [ns]')
Print(df)
The output will be:
Date
0 2021-01-01
1 2021-02-01
2 2021-03-01
Date
0 2021-01-01
1 2021-02-01
2 2021-03-01
Date datetime64 [ns]
In this example, the `'Date'` column is now of type `'datetime64 [ns]'`, which allows for date-specific operations.
Another common issue when using `astype()` is receiving a `ValueError when trying to convert a string that cannot be interpreted as a number to an integer or float. For instance:
Python
Import pandas as pd
Df = pd.DataFrame({'A': ['1', '2', 'three']})
Try: df['A'] = df['A'].astype(int)
Except ValueError as e: print(e)
The output will be:
Invalid literal for int() with base 10: 'three'
In this case, one solution is to use the `to_numeric()` function with `errors='coerce' to replace non-numeric values with `NaN`:
Python
Import pandas as pd
Df = pd.DataFrame({'A': ['1', '2', 'three']})
Df['A'] = pd.to_numeric(df['A'], errors='coerce')
Print(df)
The output will be:
A
0 1.0
1 2.0
2 NaN
In conclusion, the `astype()` function in pandas is a powerful tool for converting data types in a DataFrame. It can handle basic data types like integers and floats, as well as more complex types like datetime and categorical data types. However, it's important to understand the limitations and potential issues that may arise when using `astype()`, such as `ValueErrors` when converting invalid data types.
Honoring the Fallen: Remembering the Marine Corps' Sacrifice in Afghanistan
You may want to see also
Using 'LabelEncoder' to convert
Using LabelEncoder to convert categorical data to numbers
In machine learning, we often come across datasets with features that do not have numerical values but instead contain multiple labels. While these labels make the data more human-readable, they are incompatible with machine learning algorithms. Therefore, we must convert categorical data into numerical data. This can be done through a process called Label Encoding.
Label Encoding is a technique used to convert categorical columns into numerical ones so that they can be fitted by machine learning models, which only take numerical data. It is an important preprocessing step in a machine-learning project.
Python
Import the necessary libraries
Import pandas as pd
From sklearn.preprocessing import LabelEncoder
Set up the data
City_data = {'city_level': [1, 3, 1, 2, 2, 3, 1, 1, 2, 3],
'city_pool': ['y', 'y', 'n', 'y', 'n', 'n', 'y', 'n', 'n', 'y'],
'Rating': [1, 5, 3, 4, 1, 2, 3, 5, 3, 4],
'City_port': [0, 1, 0, 1, 0, 0, 1, 1, 0, 1],
'city_temperature': ['low', 'medium', 'medium', 'high', 'low', 'low', 'medium', 'medium', 'high', 'low']}
Convert the data into a Pandas DataFrame
Df = pd.DataFrame(city_data, columns=['city_level', 'city_pool', 'Rating', 'City_port', 'city_temperature'])
Create a function for LabelEncoder
Def Encoder(df):
# Select the columns with categorical values
ColumnsToEncode = list(df.select_dtypes(include=['category', 'object']))
# Create a LabelEncoder object
Le = LabelEncoder()
# Iterate over the columns and perform Label Encoding
For feature in columnsToEncode:
Try:
# Perform Label Encoding and assign the new values to the DataFrame
Df[feature] = le.fit_transform(df [feature])
Except:
# Print an error message if there is an issue encoding a particular feature
Print('Error encoding ' + feature)
Apply the Encoder function to the DataFrame
Df = Encoder(df)
In this example, the `city_pool` and `city_temperature` features contain categorical data. After applying the `Encoder` function, these features will be converted into numerical values. For instance, in the `city_temperature` feature, `low` may be represented by 1, `medium` by 2, and `high` by 0.
Label Encoding is a simple and straightforward approach to handling categorical data. However, it is important to note that it may not always be the best choice. One potential issue is that the numerical values can be misinterpreted by algorithms, which may assume that there is an ordinal ranking between the categories. For instance, if "Apple" is encoded as 1 and "Broccoli" is encoded as 3, the algorithm may interpret this as meaning that "Broccoli" is higher or more important than "Apple", which may not be true.
Therefore, it is important to consider other encoding techniques, such as One Hot Encoding, which can handle high-cardinality categorical variables more effectively and avoid the potential issues of misinterpretation that can arise with Label Encoding.
The Human Cost of War: Examining Taliban Fighter Casualties in Afghanistan
You may want to see also
Frequently asked questions
This error occurs when trying to convert a string to a floating-point number, and the conversion fails because the string is not a valid representation of a floating-point number.
The error may be due to the presence of non-numeric characters in the string, such as country names or other text that cannot be interpreted as a floating-point number.
To fix this error, you need to ensure that the input string contains only valid floating-point characters (digits, decimal points, and optional signs). Any non-numeric characters should be removed or replaced with numeric values before attempting the conversion.
Yes, in Python, you can use the astype() function from the NumPy library to convert an array of strings to floats. Additionally, the pandas library provides functions like pd.to_numeric() and applymap() that can handle such conversions and deal with invalid data gracefully.